technical
The datalogger is a complete multi-tier solution that includes a rich API and SD-card hardware interface (over SPI). It operates in an independent task, to be called from the main program. Requests to the logger are sent through a mailbox in a specific packet format. Currently, the logger supports read, write, delete and directory creation operations. These operations include the required mechanisms to send instructions and receive data from the SD-card. As such, given the modular nature of the project, additional operations can easily be added. In order to prevent information loss during SD-card removal, we've implemented a "safely remove" feature. SD-card connection state can be toggled using the launchpad's buttons, and is indicated by the built-in red LED (OFF signals the card is removed). While removed, packets are stored in memory.
Hardware
Required Equipment
- CC1350 Launchpad (other SoCs may be suitable).
- SD-Card breakout board.
Connecting SD-Card Breakout Board to CC1350 Launchpad
The breakout board pins (left) should be connected to the board's pins (right), by either the male or female header:
- 3.3V to 3.3V.
- GND to GND.
- CS to DIO22.
- MOSI to DIO8.
- MISO to DIO9.
- SCK to DIO10.
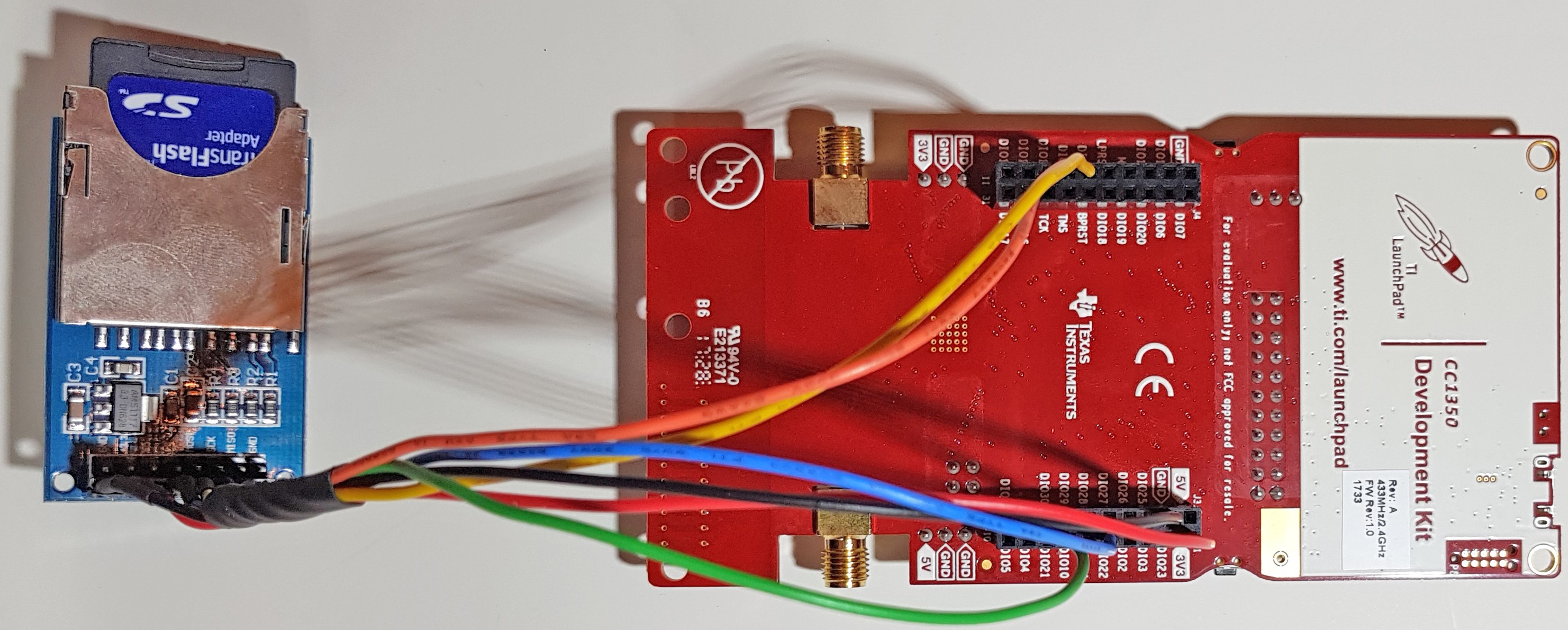
Software
Structure
DataLoggerIn addition, the logger exposes the following functions for packet creation:
- createPacket - Creates packet for logger.
- setPacket - Sets packet fields.
Handles button presses as means to toggle SD readiness ("safely remove").
FATFSHelperContains functions that are used in the logger's implementation of the FATFS API.
SDHelperHandles SD-card communication (initialization and operations).
SPIHelperContains functions that are used to communicate with the SD-card over SPI.
TimeStamp- get_formatted_fattime - Formats date to 32-bit FAT time format (2-second resolution).
Packet Structure
- opcode - Command operation code.
- data - Data to log (used for write).
- dataLen - Length of data in packet (used for R/W).
- time - Packet time-stamp in MS-DOS/FAT time format.
- filePath - Log file path (used for R/W).
Operation Codes
- RD_CMD - Read log.
- WR_CMD - Write log.
- DEL_CMD - Delete log.
- MKDIR_CMD - Create directory on SD-Card.
Time-Stamp Format
The time-stamp is formatted in 32-bit DOS/FAT time (2 seconds resolution):

A given time (year, month, day, hour, minute and second) can be formatted using the API.
challenges
The complexity of the solution posed several challenges:
Physical Connection to SD-card
The first challenge was physically connecting an SD-card to the launchpad. We experimented with different hardware solutions (e.g soldering wires to an adapter). Ultimately, reliability issues led to the use of a standard breakout board (contains pull-up resistors and bypass capacitors required for stable operation), which we wired to the female head pins of the board.
SPI transfer
In order to communicate with the connected SD-card, we had to send and receive information over SPI using Texas Instrument's non-trivial API. In the beginning, we had to use a protocol analyzer to debug the data transfers.
Communication with SD-card
On top of the physical layer, we needed to find the correct commands to operate the SD-card, as well as the specific order of initialization and other specifics.
Synchronization
The logger needs to handle requests from different tasks simultaneously. As a result, synchronization is a major factor. Unfortunately, debugging of timing issues is a severe problem in TI-RTOS. After extensive research, we decided to use the mailbox mechanism, which allows synchronization between different clients, as well as storing of packets in memory. SD availability was synchronized using a semaphore. Additionally, critical sections were defined, by disabling interrupts.
Lossless and uninterrupted transfer
Aiming to avoid unwanted data loss, we implemented a "Safely Remove" mechanism. Users can ensure no data is lost during disconnections by pressing one of the launchpad's buttons and waiting for the visual confirmation. This halts communication with the SD-card and stores the packets in memory until the button is pressed again. Removal of the SD-card requires a synchronized partial initialization in order to seamlessly resume operation.
assumptions for intended use
- It is a singleton.
- There is a maximum of one reader task.
- There is a maximum of one writer task.
Failure to meet these assumptions may cause unexpected behavior.