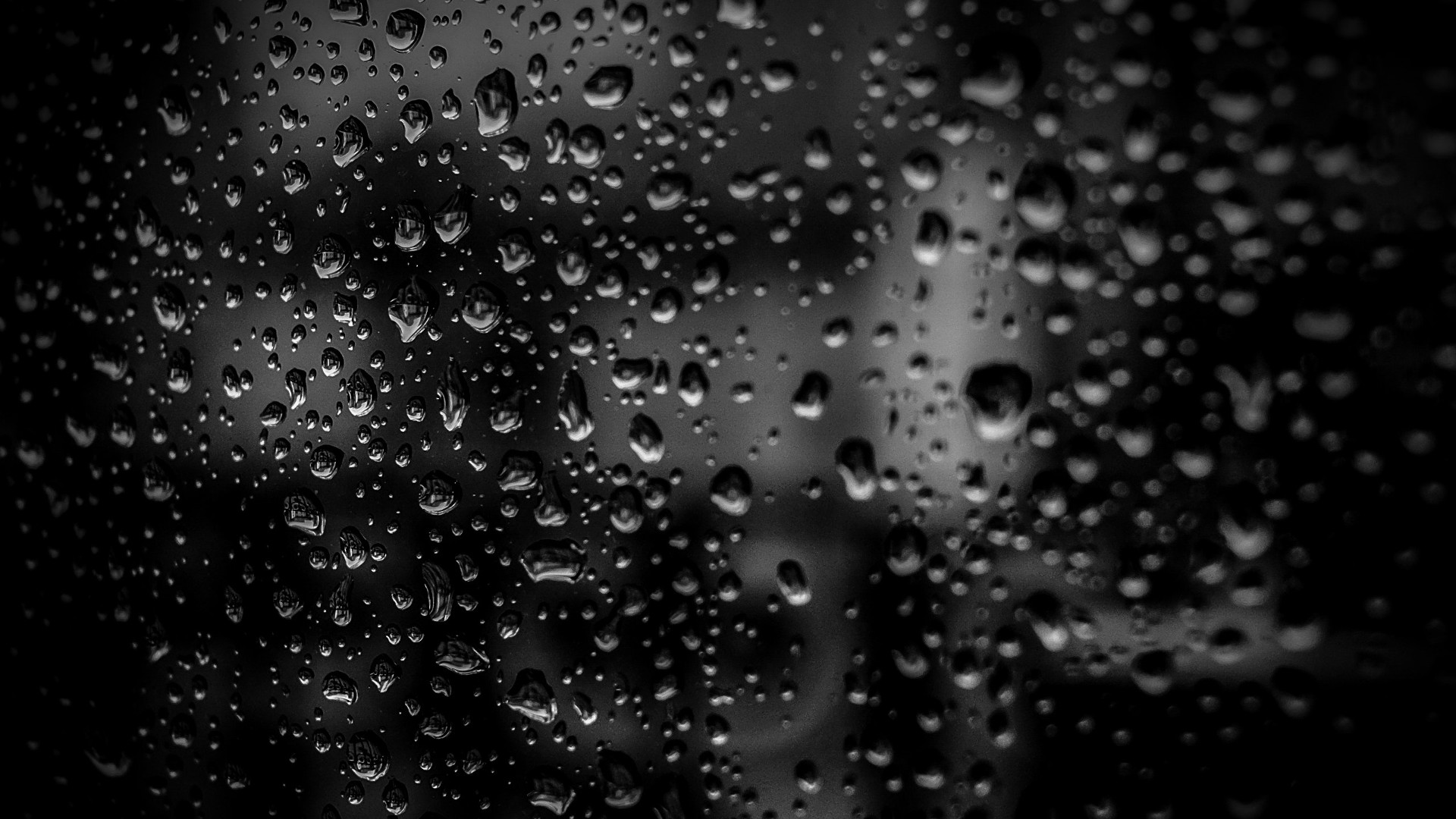

Make a fair descision about the sensitive subject that office air conditioning
The weather that makes everyone comfortable
Learn More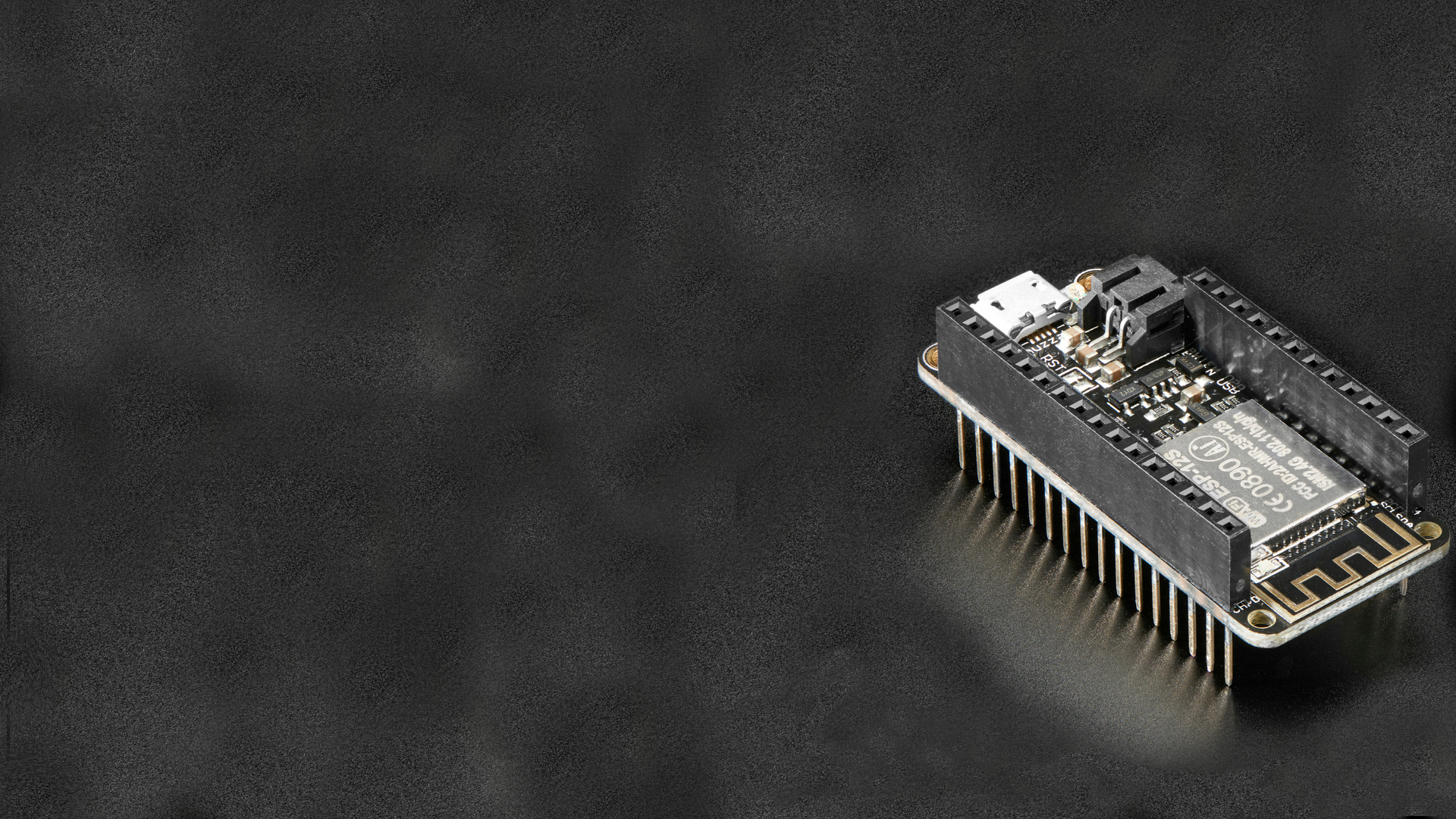
How many times have you had a disagreement with your coworkers about the air conditioner?
How many times did a signle person turn off the air conditioner for the entire lecture because he felt too cold?
The idiology behind our idea is democratic air conditioning - Every person in the room has a vote, every vote is equal, and the system decides the optimal temperature of the air conditioner based on the votes everyone cast, and the real temperature in the room.
Our solution is simple - an arduino device will be placed in each classroom/office/etc. and will be configured to work with the room's air conditioners.
Every person entering the room who has the ShAir mobile application, will be able to locate the bluetooth beacon connected to the device, allowing the user to register and vote on the room temperature.
Meanwhile, the arduino device will constantly query the server, checking if it needs to transmit a new command to the air conditioners - and if it does, will transmit the IR codes and change the temperature of the room!
The approach we took allows easy integration with existing air conditioners - installing the device is simple, only requires putting it in the room and configuring it through the administration site. No need to replace existing air conditioners or infrastructure.
Our project consists of three parts:
At the Feather HUZZAH's heart is an ESP8266 WiFi microcontroller clocked at 80 MHz and at 3.3V logic. This microcontroller contains a Tensilica chip core as well as a full WiFi stack. You can program the microcontroller using the Arduino IDE for an easy-to-run Internet of Things core.
IR radiation is radiation in frequencies outside the visible spectrum. IR is transmitted by all bodies that radiate heat. When you use any IR remote, for example a TV remote, an IR LED is used to transmit information to your TV by modulating the information on a frequency in the IR spectrum, and sending it to the TV IR receiver.
A common modulation scheme for IR communication is something called 38kHz modulation. There are very few natural sources that have the regularity of a 38kHz signal, so an IR transmitter sending data at that frequency would stand out among the ambient IR. 38kHz modulated IR data is the most common, but other frequencies can be used.
When you hit a key on your remote, the transmitting IR LED will blink very quickly for a fraction of a second, transmitting encoded data to your appliance:
Unlike TV remotes, which have a signal for each button, and maybe a "repeat" signal for long presses, AC's remotes hold state - for example, when pressing + on the fan speed, not only the button id is transmitted, but also the fact that the AC is on, whether it's in cooling or heating mode, fan speed, temperature, whether the swing is on or off, etc.
Therefore, it's not enough to record every button on the remote once- you either need to record all the possible combinations that you will want to transmit, or you will need to understand how the remote passes information and construct the signal yourself.
The temperature module, TMP_102 was connected according to it's manual specifications: to the SDA anc SCL connectors of the board.
The LED was connected to pin #16.
The arduino code consists of a few seperated modules:
Our Climate Controller uses Azure's cloud resources as a way to proccess and pass information between users and Arduino devices.
The technologies which we have used for building projects server side are:
• Azure App Service - runs our Climate Control server.
• Azure Sql Database and Server - hosts the project's DB.
• Spring - Java framework which enbales the createion of Restful API's, supports SQL server and Multithreading.
After configuring a new Climate Control device the arduino sends the server information about the tempature at a specific location where it was configured. When the server recieves this information it sends back the IR codes which the arduino needs to transmit in order tho change the rooms temperature.
While Arduino devices are transmiting information users can connect to our Climate Control server, get the real tempature and place their own vote to affect the overall rooms temperature.
All data passed to or from server is encrypted as it uses HTTPS (Azure configuration). Sql injection is prevented by the use of prepared statements with variable binding. Moreover, Azure Sql Server Firewall rejects all connections to the server and the databases except for connections from other Azure services.
Our project uses Microsoft Sql Server which is a relational database management system.
Our DB structure:
Gets all the AC’s configured supported by ShAir
Configures the device location and connected AC’S
Receives device data, processes it and send back users requests and AC codes
Connects a new user to ShAir Server and sends in response users location data
Handles users change temperature request
Sends user his current location by BT device ID
Send user temperature and location data
Enables user to disconnect from ShAir Server
This project was submitted by Daniel S., Inbal M. and Arthur V.
At the time of submission we are third year students in Tel Aviv Unitersity, studying for BsC in Computer Science.
September 4th, 2018.
© Designed and Developed by UIdeck